Loop Statements/Iteration Statemens in Java:
while, do-while, for
these three statements are used as a commands repeater statement called as loop/iteration statements.
All commands within loop statement, runs repeatedly until given condition is true.
****************************
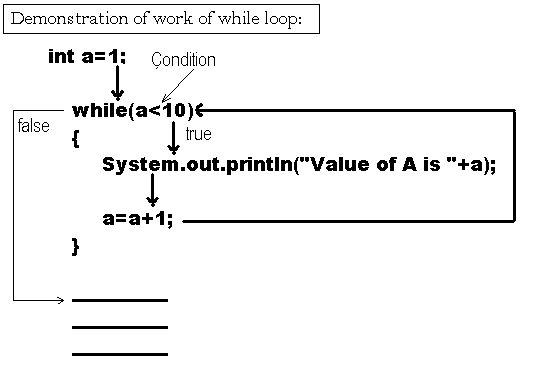
eg.
//example of while loop
class whil {
public static void main(String ar[]) {
int a=1;
while(a<10)
{
System.out.println("Value of A is "+a);
a=a+1;
}//end of while
}//end of main
}//end of class
to compile :
c:\myjavaprg>javac whil.java
to run :
c:\myjavaprg>java whil
Output:
Value of A is 1
Value of A is 2
Value of A is 3
Value of A is 4
Value of A is 5
Value of A is 6
Value of A is 7
Value of A is 8
Value of A is 9
****************************
* do-while loop statement
****************************
regardless condition, statements in do while loop executes first, then
it checks wheather given condition is true or not
if condition is true then loop executes commonds again and again till condition is true.
if condition is not true then loop ends.
eg.
//example of do-while loop
class dowhil {
public static void main(String ar[]) {
int a=1,b=11;
do
{
System.out.println("Value of A is "+a); //first prints value of variable A
a=a+1; //increments value of variable A
} while(a<10); //checks value of A is less 10 or not if yes goes to do statement
do
{
System.out.println("\n\nValue of B is "+b); //first prints value of variable B
b=b+1; //increments value of variable B
} while(b<10); //checks wheather B is less 10 if not end do statement
//even if condition of do while is not true at first step then also commands withing do while gets run
}//end of main
}//end of class
to compile :
c:\myjavaprg>javac dowhil.java
to run :
c:\myjavaprg>java dowhil
Output:
Value of A is 1
Value of A is 2
Value of A is 3
Value of A is 4
Value of A is 5
Value of A is 6
Value of A is 7
Value of A is 8
Value of A is 9
Value of B is 11
*****************
for loop
*****************
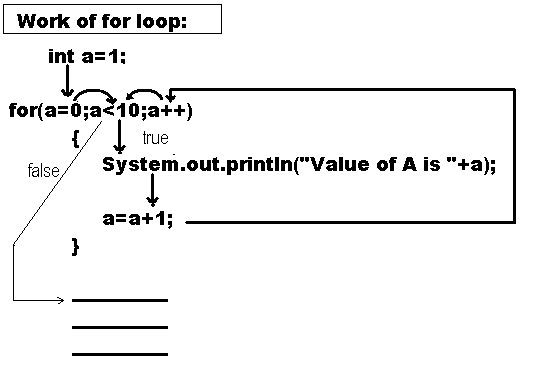
//example of for loop
class forloop {
public static void main(String ar[]) {
int i=1;
//first assigns value 1 to i,
//then checks wheather i is less than 10,
//if yes prints value of i
//then increments value of i by one
//again checks wheather incremented value of i is less than 10
//if yes print, increment, check till value is less than 10
//if value is not less than 10, i.e. equal to 10 or greater than 10 then
//for loop stops execution of commands in it and transfer control to first statement outside of "for loop".
//in for loop, initilisation, condition, increment/decrements these three important statements group in one line.
//so there are very little chance to omit one of them
for(i=1;i<10;i++)
{
System.out.println("Value of i: "+i); //prints value of variable i
} //end of for loop
}//end of main
}//end of class
to compile :
c:\myjavaprg>javac forloop.java
to run :
c:\myjavaprg>java forloop
Output:
Value of i: 1
Value of i: 2
Value of i: 3
Value of i: 4
Value of i: 5
Value of i: 6
Value of i: 7
Value of i: 8
Value of i: 9