//program using if statement
class ife {
public static void main(String ar[]) {
int month=2,year=2016;
int days=0;
if((month == 2) && ((year % 4)==0)) days = 29; //find if given year is leap year
if((month == 2) && ((year % 4)!=0)) days = 28; //f given year is not leap year
if(month == 1 || month == 3 || month == 5 || month == 7 || month == 8 ||month == 10 ||month == 12)
days = 31;
if(month == 4 || month == 6 || month == 9 || month == 11) //months has 30 days in it
days = 30;
System.out.println("Month="+month+" year="+year+" has "+days+" days");
}
}
to compile :
c:\myjavaprg>javac ife.java
to run :
c:\myjavaprg>java ife
Output:
Month=2 year=2016 has 29 days
SWITCH Statement
============
It is a better way to represent multi if...else...if...else... statements.
It checks value of expression which is given in bracket of switch statement.
syntax is :
switch (exp)
{
case value1:
some valid java statements
break;
case value2:
some valid java statements
break;
case value3:
some valid java statements
break;
.
.
.
default:
some valid java statements
}
break statement is optional.
If you not use break statement then execution is on going with other cases
till break statement occurs or end of switch statement.
You can use switch statement in between outer switch statement (nesting is allowed).
eg.
//example of switch statement
class swit {
public static void main(String ar[]) {
int i = 7;
switch(i)
{
case 1: System.out.println("Sunday");
break;
case 2: System.out.println("Monday");
break;
case 3: System.out.println("Tuesday");
break;
case 4: System.out.println("Wedensday");
break;
case 5: System.out.println("Thursday");
break;
case 6: System.out.println("Friday");
break;
case 7: System.out.println("Saturday");
break;
default: System.out.println("No such day!");
}
}
}
to compile :
c:\myjavaprg>javac swit.java
to run :
c:\myjavaprg>java swit
Output:
Saturday
another form of switch statement:
//example of switch statement
class swit1 {
public static void main(String ar[]) {
int i = 5;
switch(i)
{
case 11:
case 12:
case 1:
case 2: System.out.println("Its Winter");
break;
case 3:
case 4:
case 5:
System.out.println("oh no!! Summer");
break;
case 6:
case 7:
case 8:
case 9:
System.out.println("Aah! Rainy days");
break;
case 10:
System.out.println("October Heat!");
break;
}
}
}
to compile :
c:\myjavaprg>javac swit1.java
to run :
c:\myjavaprg>java swit1
Output:
oh no!! Summer
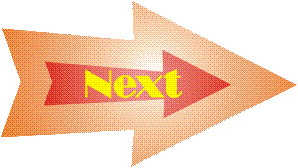