You must set path variable to compile and run java programs as follows:
PATH=C:\WINDOWS\system32;C:\WINDOWS;C:\WINDOWS\System32\Wbem;C:\Program Files\Java\jdk1.7.0_25\bin
then must restart your computer.
to run java program type :
c:\myjavaprog>javac hello.java
The javac compiler creates a file called hello.class that contains the bytecode version of the program.
The java bytecode is the intermediate representation of your progrtam that contains instuction the java interpreter will execute.
So the output of javac, bytecode is not code that can be directly executed.
To run the java program, you must use the java interpreter, called java.exe.
c:\myjavaprog>java hello
or to take a output into a file:
c:\myjavaprog>java hello > fl.txt
Bitwise Logical Operators
=========================
Bitwise AND (&)
Input1 & Input2 Output
1 1 1
1 0 0
0 1 0
0 0 0
101 5
& 110 6
---
100 4
---------------------------------------------------
Bitwise OR (|) Pipe symbol near backspace key.
Input1 | Input2 Output
1 1 1
1 0 1
0 1 1
0 0 0
101 5
| 110 6
---
111 7
---------------------------------------------------
Bitwise XOR (^)
Input1 ^ Input2 Output
1 1 0
1 0 1
0 1 1
0 0 0
101 5
^ 110 6
---
011 3
---------------------------------------------------
Bitwise NOT (~)
Input Output
1 0
0 1
---------------------------------------------------
//Bitwise logical operator
class bl {
public static void main(String a[]) {
int l,m,n;
l=5; m=6;
/*bitwise and
101 5
& 110 6
---
100 4
*/
n=l & m;
System.out.println("5 & 6 = "+n);
/*bitwise or
101 5
| 110 6
---
111 7
*/
n=l | m;
System.out.println("5 | 6 = "+n);
/*bitwise xor
101 5
^ 110 6
---
011 3
*/
n=l ^ m;
System.out.println("5 ^ 6 = "+n);
//bitwise not
//0000000000000000 0000000000000101 = 5
//1111111111111111 1111111111111010 = 4294967290
//bitwise NOT operation exceeds limit of int = 2147483647
n = ~l;
System.out.println("~5 = "+ n);
}
}
compile program
c:\myjavaprog>javac bl.java
run program
c:\myjavaprog>java bl
output
5 & 6 = 4
5 | 6 = 7
5 ^ 6 = 3
~5 = -6
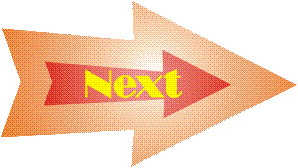